1. Preface¶
1.1. Topstx Install¶
sudo pip3 install topstx-2.4.1-cp36-cp36m-linux_x86_64.whl #or other version
1.2. Revision History¶
Version |
Description |
Date |
---|---|---|
V1.0 |
Initial version |
2023.04 |
1.3. annotate¶
The annotate()
function annotates a code range, i.e., one or more statements.
Each code range may have a message and a color associated with it.
This makes it easy to distinguish ranges when visualizing them.
annotate
can be used in two ways:
As a decorator:
@topstx.annotate(message="my_message", color="blue")
def my_func():
pass
As a context manager:
with topstx.annotate(message="my_message", color="green"):
pass
When used as a decorator, the message
argument defaults to the
name of the function being decorated:
@topstx.annotate() # message defaults to "my_func"
def my_func():
pass
1.4. start_range and end_range¶
In certain situations, it is impossible to use annotate()
,
e.g., when a code range spans multiple functions or in asynchronous code.
In such cases, the start_range()
and end_range()
functions
can be used instead.
The start_range()
function is called at the beginning of a code range,
and returns a handle. The handle is passed to the end_range()
function,
which is called at the end of the code range.
rng = topstx.start_range(message="my_message", color="blue")
# ... do something ... #
topstx.end_range(rng)
1.5. mark¶
The mark()
function marks an instantaneous event in the execution of a program.
For example, you may want to mark when an exceptional event occurs:
try:
something()
except SomeError():
topstx.mark(message="some error occurred", color="red")
# ... do something else ...
1.6. Domains¶
In addition to a message and a color, annotations can also have a domain associated with them. This allows grouping annotations.
import time
import Topstx
@topstx.annotate(color="blue", domain="Domain_1")
def func_1():
time.sleep(1)
@topstx.annotate(color="green", domain="Domain_2")
def func_2():
time.sleep(1)
@topstx.annotate(color="red", domain="Domain_1")
def func_3():
time.sleep(1)
func_1()
func_2()
func_3()
Domains should be used sparingly as they are expensive to create. It is typically recommended to use a single domain per library.
1.7. Categories¶
Categories allow grouping of annotations within a domain.
import time
import topstx
@topstx.annotate(color="blue", domain="Domain_1", category="Cat_1")
def func_1():
time.sleep(1)
@topstx.annotate(color="green", domain="Domain_1", category="Cat_2")
def func_2():
time.sleep(1)
@topstx.annotate(color="red", domain="Domain_2", category="Cat_1")
def func_3():
time.sleep(1)
@topstx.annotate(color="red", domain="Domain_2", category=2)
def func_4():
time.sleep(1)
func_1()
func_2()
func_3()
func_4()
In the example above, func_1 and func_2 are grouped under the domain Domain1, but under different categories within that domain.
Although func_1 and func_3 are both grouped under a category named Cat_1, they are unrelated as each domain maintains its own categories.
Unlike domains, categories are not expensive to create and manage. Thus, you should prefer categories for maintaining several groups of annotations.
2. Automatic function annotation¶
Annotating code manually is not always desirable, for example, when you have lots of functions to annotate, or when you want to capture information from third-party libraries.
Topstx
can automatically annotate each function call in your program.
Note that doing this adds a tiny amount of
overhead to each and every function invocation, which can significantly
impact the overall runtime (by more than 10x).
This can give you lots of useful information that manual annotation cannot
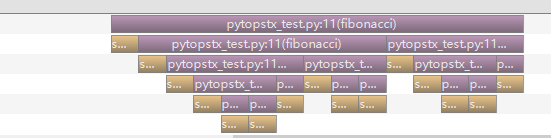
2.1. Command-line interface¶
You can invoke Topstx
as a command-line script, which annotates every function call,
with no changes to the source code:
python -m Topstx script.py
2.2. The Profile class¶
You can also use Profile
to enable and disable
automatic function annotation in different parts of
your program:
pr = topstx.Profile()
pr.enable() # begin annotating function calls
# -- do something -- #
pr.disable() # stop annotating function calls
3. Topstx API Reference¶
3.1. Topstx¶
Profile¶
def Profile()
Returns
- handle
A Profile handle
annotate¶
The annotate() function annotates a code range
def annotate(name=None,domain=None,category=None,color=None)
mark¶
The mark() function marks an instantaneous event in the execution of a program.
def mark(name,domain=None,category=None,color=None)
start_range¶
The start_range() function is called at the beginning of a code range, and returns a handle.
def start_range(name,domain=None,category=None,color=None)
Parameters
- name
input. the name of the trace
- domain
input. the domain of the trace
Returns
- range_id
range_id use to call end_range
end_range¶
The end_range() function is called at the end of the code range
def end_range(range_id)
Parameters
- range_id
input. the range_id of the trace return by start_range
push_range¶
The push_range() function is called at the beginning of a push/pop range.
def push_range(name,domain=None,category=None,color=None)
Parameters
- name
input. the name of the trace
- domain
input. the domain of the trace
pop_range¶
The pop_range() function is called at the end of the push/pop range
def pop_range(range_id)
3.2. Profile¶
enable¶
Start annotating function calls automatically.
def enable()
disable¶
Stop annotating function calls automatically.
def disable()